Parameter Sniffing and Conditional Logic in SQL Server Stored Procedures
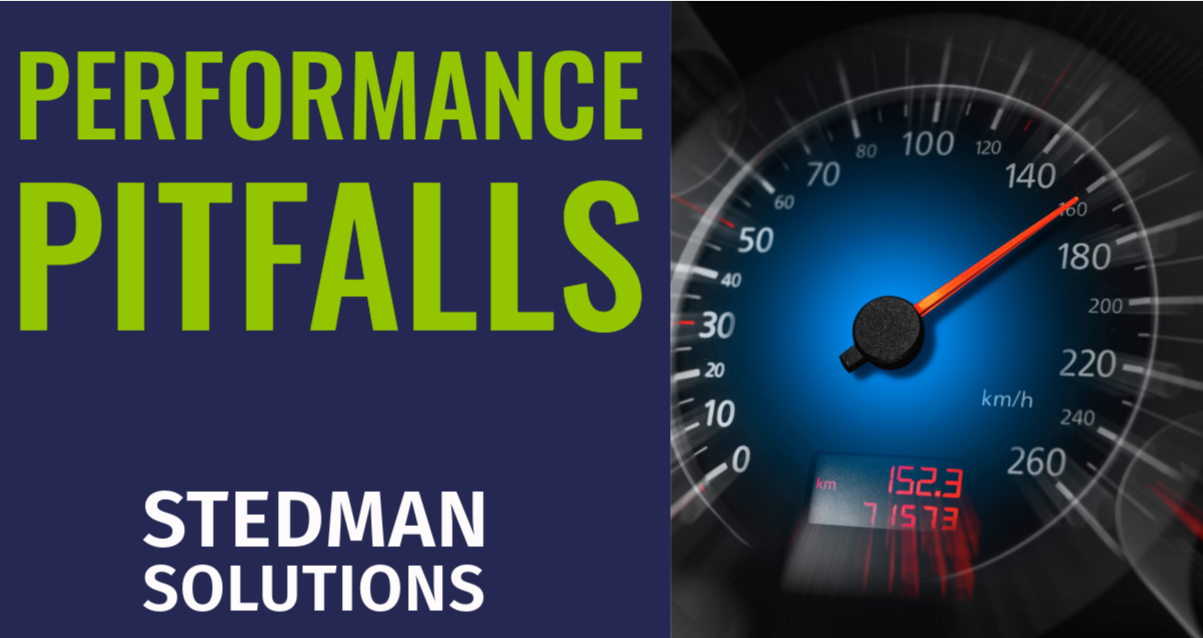
Parameter Sniffing and Conditional Logic in SQL Server Stored Procedures: A Performance Pitfall
Parameter sniffing is a well-known feature in SQL Server that can significantly impact query performance. While it often leads to optimized query execution, it can sometimes cause performance issues, especially when combined with conditional logic in stored procedures. In this article, we’ll explore how parameter sniffing interacts with conditional logic and how this interaction can lead to suboptimal performance.
Understanding Parameter Sniffing
Parameter sniffing occurs when SQL Server generates an execution plan for a stored procedure or parameterized query based on the parameter values provided during the first execution. This execution plan is then cached and reused for subsequent executions, potentially leading to performance problems if the initial parameters are not representative of typical usage.
The Role of Conditional Logic
Stored procedures often contain conditional logic to handle different scenarios. For example:
CREATE PROCEDURE GetOrders @CustomerId INT = NULL, @OrderDate DATE = NULLASBEGIN IF @CustomerId IS NOT NULL BEGIN SELECT OrderId, OrderDate, TotalAmount FROM Orders WHERE CustomerId = @CustomerId; END ELSE IF @OrderDate IS NOT NULL BEGIN SELECT OrderId, OrderDate, TotalAmount FROM Orders WHERE OrderDate = @OrderDate; END ELSE BEGIN SELECT OrderId, OrderDate, TotalAmount FROM Orders; ENDEND
In this example, the stored procedure handles three different scenarios based on the provided parameters.
How Parameter Sniffing Affects Conditional Logic
When SQL Server first compiles the stored procedure, it generates an execution plan based on the values of the parameters used during the initial execution. This means that only the branch of the conditional logic that matches the initial parameters is used to create the execution plan. Subsequent executions reuse this plan, which might not be optimal for other branches of the logic.
Example Scenario
- First Execution with
@CustomerId
: - Subsequent Execution with
@OrderDate
:
EXEC GetOrders @CustomerId = 1;
SQL Server generates an execution plan optimized for the query:
SELECT OrderId, OrderDate, TotalAmountFROM OrdersWHERE CustomerId = @CustomerId;
This plan might use an index seek on the CustomerId
column.
EXEC GetOrders @OrderDate = '2023-01-01';
SQL Server reuses the previously cached plan. Since the plan was optimized for CustomerId
, it may not perform well for the OrderDate
scenario, potentially resulting in inefficient execution.
Performance Issues Caused by Conditional Logic and Parameter Sniffing
The primary issue is that the cached execution plan is not tailored for the different query patterns encapsulated within the conditional logic. This can lead to:
- Suboptimal Index Usage: An execution plan optimized for a selective
CustomerId
query might not efficiently handle a less selectiveOrderDate
query. - Increased I/O and CPU Usage: Plans not suited for the data distribution can lead to increased I/O operations and CPU usage, slowing down query performance.
- Inconsistent Performance: Different branches of the conditional logic might experience inconsistent performance depending on the cached plan’s suitability.
Mitigation Strategies
To mitigate performance issues caused by parameter sniffing in stored procedures with conditional logic, consider the following strategies:
1. Use OPTION (RECOMPILE)
Force SQL Server to recompile the execution plan for each execution, ensuring that the plan is optimized for the current parameter values.
CREATE PROCEDURE GetOrders @CustomerId INT = NULL, @OrderDate DATE = NULLASBEGIN IF @CustomerId IS NOT NULL BEGIN SELECT OrderId, OrderDate, TotalAmount FROM Orders WHERE CustomerId = @CustomerId OPTION (RECOMPILE); END ELSE IF @OrderDate IS NOT NULL BEGIN SELECT OrderId, OrderDate, TotalAmount FROM Orders WHERE OrderDate = @OrderDate OPTION (RECOMPILE); END ELSE BEGIN SELECT OrderId, OrderDate, TotalAmount FROM Orders OPTION (RECOMPILE); ENDEND
2. Separate Procedures
Create separate stored procedures for each query pattern, ensuring that each procedure is optimized for its specific scenario.
CREATE PROCEDURE GetOrdersByCustomerId @CustomerId INTASBEGIN SELECT OrderId, OrderDate, TotalAmount FROM Orders WHERE CustomerId = @CustomerId;ENDCREATE PROCEDURE GetOrdersByOrderDate @OrderDate DATEASBEGIN SELECT OrderId, OrderDate, TotalAmount FROM Orders WHERE OrderDate = @OrderDate;END
3. Dynamic SQL
Use dynamic SQL to generate and execute the appropriate query at runtime, ensuring that the execution plan is always tailored for the specific parameter values.
CREATE PROCEDURE GetOrders @CustomerId INT = NULL, @OrderDate DATE = NULLASBEGIN DECLARE @SQL NVARCHAR(MAX); IF @CustomerId IS NOT NULL BEGIN SET @SQL = N'SELECT OrderId, OrderDate, TotalAmount FROM Orders WHERE CustomerId = @CustomerId'; EXEC sp_executesql @SQL, N'@CustomerId INT', @CustomerId; END ELSE IF @OrderDate IS NOT NULL BEGIN SET @SQL = N'SELECT OrderId, OrderDate, TotalAmount FROM Orders WHERE OrderDate = @OrderDate'; EXEC sp_executesql @SQL, N'@OrderDate DATE', @OrderDate; END ELSE BEGIN SET @SQL = N'SELECT OrderId, OrderDate, TotalAmount FROM Orders'; EXEC sp_executesql @SQL; ENDEND
4. Plan Guides
Use plan guides to enforce specific execution plans for different branches of the logic.
Conclusion
Parameter sniffing can significantly impact the performance of stored procedures that use conditional logic. Understanding how SQL Server generates and caches execution plans can help you identify potential performance issues and implement strategies to mitigate them. By using techniques like recompiling queries, separating procedures, using dynamic SQL, or applying plan guides, you can ensure more consistent and optimized query performance.
For more insights into SQL Server Performance tuning, consider exploring the Database Health Monitor, a comprehensive tool designed to help you monitor and optimize your SQL Server instances. And if you need expert assistance, Stedman Solutions offers Managed Services to help you maintain peak performance and reliability in your SQL Server environments.
Getting Help from Steve and the Stedman Solutions Team
We are ready to help. Steve and the team at Stedman Solutions are here to help with your SQL Server needs. Get help today by contacting Stedman Solutions through the free 30 minute consultation form.
Leave a Reply