Queries Needing Params
Why Queries Need Parameters and the Power of Parameterized Queries in SQL Server
SQL Server is a versatile database platform that provides robust functionality for managing and querying data. However, crafting queries effectively can be the difference between a secure, high-performing application and one that’s vulnerable to SQL injection attacks or plagued by inefficiencies.
This blog post delves into why queries need parameters, the benefits of using parameterized queries, and how to implement them effectively in SQL Server.
Why Queries Need Parameters
When crafting SQL queries, especially in applications, it’s common to accept user input to filter data or perform specific operations. For instance, consider this simple query:
SELECT * FROM Customers WHERE CustomerID = 'C123';
Now imagine a scenario where the CustomerID
value is dynamically passed by the user. If not handled correctly, this approach can lead to significant problems:
1. SQL Injection Risks
Without proper parameterization, directly concatenating user inputs into a query can expose your application to SQL injection attacks. For example, a malicious user might enter:
C123'; DROP TABLE Customers; --
The resulting query would be:
SELECT * FROM Customers WHERE CustomerID = 'C123'; DROP TABLE Customers; --';
This query could delete your Customers
table—a catastrophic security failure.
2. Poor Query Plan Reuse
Queries without parameters are treated as unique by SQL Server, even if the structure is identical but the input values differ. This forces SQL Server to compile a new execution plan for each query, wasting resources and reducing performance.
What Are Parameterized Queries?
A parameterized query separates SQL code from data inputs, passing the data as parameters instead of embedding it directly into the query string. For example:
SELECT * FROM Customers WHERE CustomerID = @CustomerID;
Here, @CustomerID
is a parameter, and the actual value is supplied separately by the application or the query execution framework.
Benefits of Parameterized Queries
1. Enhanced Security
Parameterized queries prevent SQL injection by treating user inputs as data, not executable code. SQL Server ensures the inputs cannot alter the query structure, safeguarding your database.
2. Improved Query Plan Reuse
By using parameters, SQL Server reuses execution plans for similar queries, reducing the need for constant query recompilation. This results in better CPU efficiency and faster query execution.
3. Readability and Maintainability
Parameterized queries are cleaner and easier to read compared to dynamically constructed SQL strings. This improves code maintainability and debugging.
4. Data Type Validation
When parameters are explicitly defined, SQL Server validates their types, reducing the chances of data-related errors.
How to Use Parameterized Queries in SQL Server
1. Using Parameters in T-SQL
You can explicitly define and use parameters in T-SQL queries. For example:
DECLARE @CustomerID NVARCHAR(10);
SET @CustomerID = 'C123';
SELECT * FROM Customers WHERE CustomerID = @CustomerID;
2. Parameterized Queries in Applications
Modern application frameworks like .NET, Python, or Java support parameterized queries natively. For example, in .NET using C#:
using (SqlConnection connection = new SqlConnection(connectionString))
{
string query = "SELECT * FROM Customers WHERE CustomerID = @CustomerID";
SqlCommand command = new SqlCommand(query, connection);
command.Parameters.AddWithValue("@CustomerID", customerID);
connection.Open();
SqlDataReader reader = command.ExecuteReader();
while (reader.Read())
{
Console.WriteLine(reader["CustomerName"]);
}
}
3. Dynamic SQL with sp_executesql
When constructing dynamic SQL in SQL Server, use sp_executesql
with parameters to prevent SQL injection and enable plan reuse. Example:
DECLARE @Query NVARCHAR(MAX);
DECLARE @CustomerID NVARCHAR(10) = 'C123';
SET @Query = 'SELECT * FROM Customers WHERE CustomerID = @CustomerID';
EXEC sp_executesql @Query, N'@CustomerID NVARCHAR(10)', @CustomerID;
Common Mistakes to Avoid
1. Using AddWithValue
Incorrectly
While AddWithValue
is convenient, it can sometimes lead to implicit conversions if the parameter’s type does not match the column’s data type. Always specify data types explicitly if performance is a concern.
2. Concatenating Parameters
Avoid concatenating parameters into the SQL string, as it defeats the purpose of parameterization and reintroduces vulnerabilities.
3. Ignoring Query Plan Cache
Even with parameterized queries, excessive diversity in parameters (e.g., using unique values that rarely repeat) can lead to plan cache bloat. Monitor your query plan cache regularly.
Real-World Example
At Stedman Solutions, we encountered a client struggling with performance issues caused by dynamically constructed SQL queries. Their application generated dozens of nearly identical queries with varying values, leading to high CPU usage and slow response times.
By refactoring their queries to use parameters and leveraging sp_executesql
, we reduced query compilation time by 40% and improved overall application performance. Additionally, this approach eliminated SQL injection risks, giving the client peace of mind about their database security.
Conclusion
Parameterized queries are a cornerstone of secure and efficient SQL Server development. By separating SQL code from user inputs, you enhance security, improve query performance, and simplify maintenance. Whether you’re working in T-SQL or within an application, adopting parameterized queries is a best practice you can’t afford to ignore.
If you’re dealing with SQL Server performance issues or need help refactoring your queries, Stedman Solutions can help. Our Managed Services ensure your database is secure, efficient, and optimized for your business needs. Contact us today to get started!
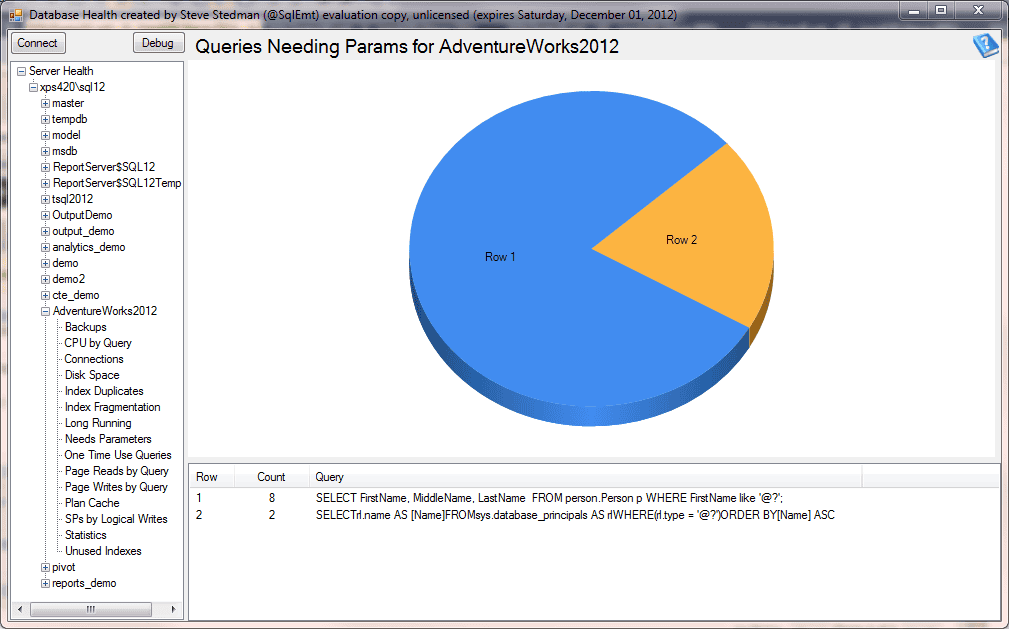
From here you can click through to the Queries Needing Params Advisor for additional details.
You get to the Queries Needing Params page from the Database Overview Page or from the hierarchy tree.
Enroll Today!
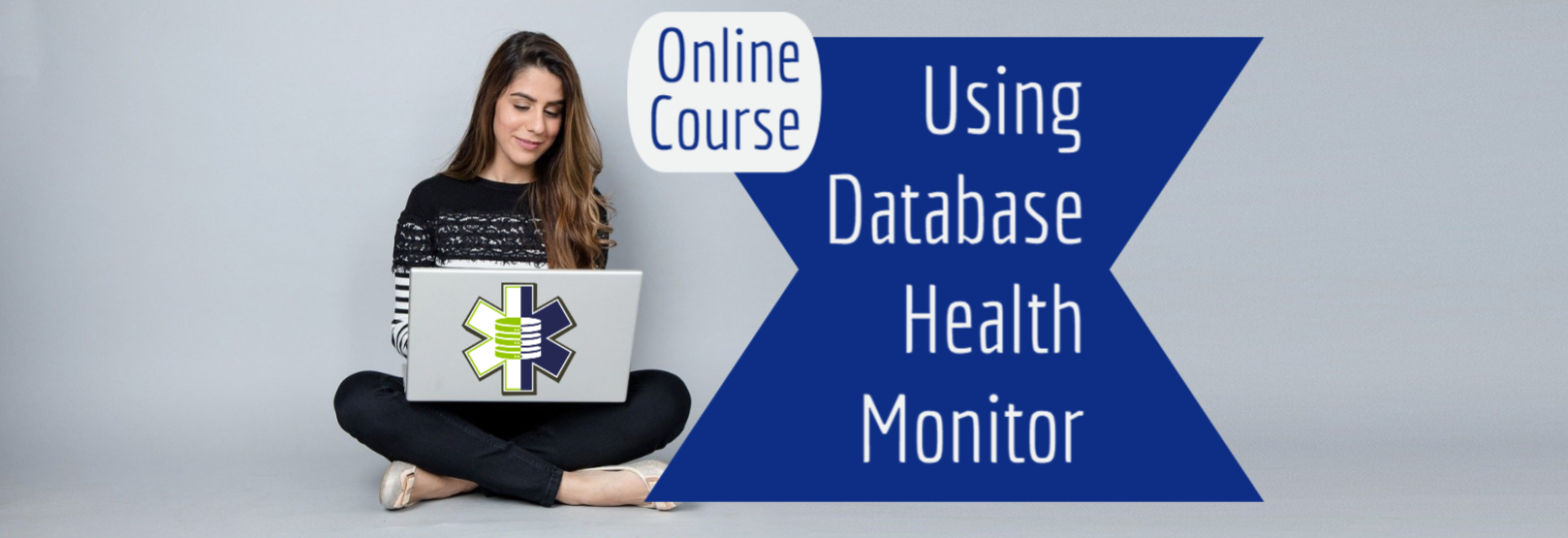

Steve and the team at Stedman Solutions are here for all your SQL Server needs.
Contact us today for your free 30 minute consultation..
We are ready to help!
Contact Info
Stedman Solutions, LLC.PO Box 3175
Ferndale WA 98248
Phone: (360)610-7833
Leave a Reply